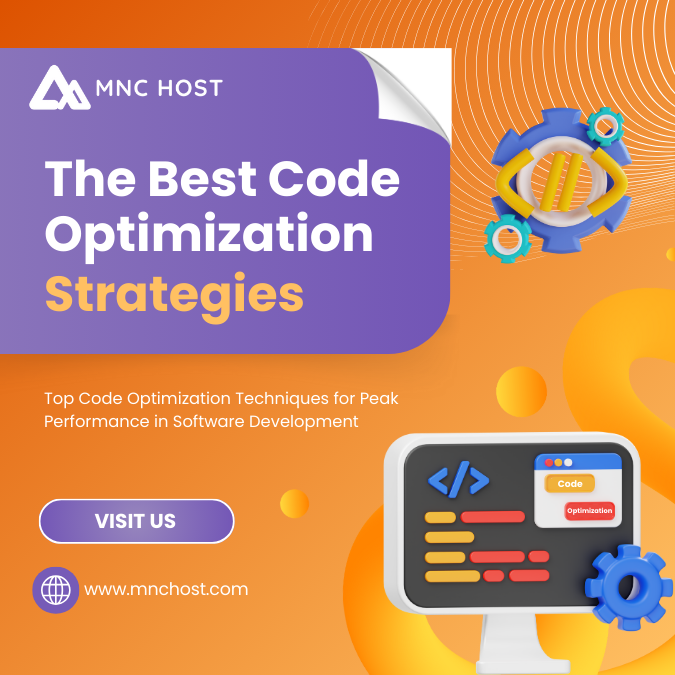
In the context of writing software, one of the most important characteristics of a soft ware system is its performance. Code optimization is the procedure by which the code is made more efficient and performs well without any modifications. Reduction in time complexity, space or memory complexity or other resource consumption in a program is known as. In this article, we will explore what we consider to be some of the best practices for code optimization that developers can use to improve the performance of their software.
- The advanced technique and tool for the most efficient algorithms and data structures. The selection of certain algorithms or data structures may greatly affect the efficiency of a program you are going to write. Here are a few tips:Here are a few tips:
- Algorithm Complexity: Try to use the algorithms with the lower time complexity wherever possible. For example, if there is time comparison between two sorting algorithms, one being O(n log n) such as Merge Sort or Quick Sort, the other being O(n^2) such as Bubble Sort, then the first is generally preferable if n is large.
- Appropriate Data Structures: This here is why it is said data structure should match the kind of tasks you want to solve with your data. For instance, the hash tables such as dictionaries in the Python or HashMap in Java can provide an average time complexity of O(1) for lookups unlike lists or arrays with time of O(n).
- Code Profiling Profiling enables one to know the places in the code that are possibly using a lot of CPU or memory space. Tools like Python per profile, Java Visual virtual machine, and Node. js has a profiler built-in as well and it can help you identify where the major bottlenecks are.
- Focus on Hotspots: Once you know them, then target optimizing these areas of high traffic and activity levels. In general, the approach followed is that 80% of the system’s performance improvement can be achieved by working on 20% of the most critical code (Pareto Principle).
- Measure Before and After: It is important to also always take a pre and post assessment of the various changes made to ensure that the optimizations made do work.
- Minimizing I/O Operations Many I/O operations like disk read or write, and network communication among others, are slow and constitute raw performance inhibitors.
- Batch Processing: Don’t process data one item by one; try to process data in bigger sets, in lots. He said this minimizes on the overhead that may be brought about by many cycles of I/O operations.
- Asynchronous I/O: When possible, use the asynchronous read/write I/O calls which do not block the main thread. In languages like Python, there are certain libraries such as asyncio that can be utilised in order to carry out asynchronous I/O.
- Memory Management It is necessary constantly to focus on memory optimization as it takes a severe toll on the performance. Memory usage when it is overused leads to swapping and at certain point slows down the system.
- Avoid Memory Leaks: Make sure you are not erroneously running out of memory or having memory swapped out unexpectedly. For instance in languages such as C++ memory can be automatically managed by the use of smart pointers.
- Garbage Collection: In languages that use garbage collectors (ala Java, Python etc). understanding the garbage collector and how to tweak it for better performance is useful. For example, to reduce the rates of garbage collection, it is possible to minimize the number of temporary objects.
- The two techniques are inline functions and loop unrolling. Inlining functions and unrolling loops are common optimization techniques:Inlining functions and unrolling loops are common optimization techniques:
- Inline Functions: Local functions in other cases serve as candidates for inlining to minimize the cost caused by function call. Most compiler provide options that would allow the automatic inlining of functions.
- Loop Unrolling: If there are loops that run frequently numerous means of unrolling a loop can help decrease the overhead of loop control while at the same time aid in the performance of tight loops. Although this has the potential to create larger code size, which should be averted, it can be really helpful.
- Parallelism and Concurrency Utilizing multiple cores effectively can significantly improve performance:Utilizing multiple cores effectively can significantly improve performance:
- Multi-threading: Apply multi-threading when you want to execute more operations at the same time. But race conditions as well as deadlocks can present a problem here.
- Parallel Processing: In languages such as Python the multiprocessing libraries can be employed to parallelising concepts and therefore, leverage multi-core processors.
- Avoiding Premature Optimization While optimization is important, it’s crucial to avoid premature optimization:While optimization is important, it’s crucial to avoid premature optimization:
- Maintain Readability: If a code is optimized well, its readability is sometime a problem and maintaining the code also becomes a tedious task. Make sure your code does not become an unmanageable mess.
- Optimize When Necessary: He thinks that readability or clarity of code and correctness are more important than performance of the code. It is always preferable to optimize when one is troubleshooting for performance problems about the application.
- Using Compiler Optimizations Modern compilers can perform various optimizations to improve performance:Modern compilers can perform various optimizations to improve performance:
- Optimization Flags: Make use of such flags that are offered by your compiler optimizer. For example, GCC has the flags such as -O2 and -O3 to facilitate different levels of optimization.
- Profile-Guided Optimization (PGO): PGO applies information gained from profiling to fine-tune code fragments that are more commonly, executed.
- Reducing Function Call Overheads Function calls can introduce overhead, especially in performance-critical sections of the code:Function calls can introduce overhead, especially in performance-critical sections of the code:
- Inline Small Functions: Inlining small functions removes the cost of the call overhead For the purpose of this context, we have previously discussed about it.
- Reduce Recursive Calls: If a given function is highly recursive then it should be flattened if it is possible to do so.
- Caching Caching is a technique where results of expensive computations are stored so that future requests can be served faster:Caching is a technique where results of expensive computations are stored so that future requests can be served faster:
- Memoization: Recursion also has memoization where in algorithms, it can store costly calls and their results hence avoiding repetition.
- Database Caching: Use in-memory storages such as Redis to cache the frequently used database queries so that the databases are not loaded and response time is faster.
- Optimize Data Access Patterns The way data is accessed can affect performance, especially in large applications:The way data is accessed can affect performance, especially in large applications:
- Locality of Reference: Make use of data locality by keeping data that are usually accessed at the same time stored at the same place to help the CPU cache. This concept goes by the name of spatial locality.
- Prefetching: At times pre-loading of data, prior to its use is possible and might be beneficial in minimizing cache misses.
- String and Regex Optimization String manipulations and regular expressions can be resource-intensive:String manipulations and regular expressions can be resource-intensive:
- Immutable Strings: To note, in languages where the string is frozen (as, for example, in Java and Python), the use of concatentation may be really slow. Choose string builders or other related constructs to work with concatenation.
- Optimized Regex: If a regular expression is to be used multiple times it should be compiled first. It is also important that one does not use complicated regexes that can in fact be simplified.
13. Network Optimization For applications involving network communication, optimizing network usage is crucial:For applications involving network communication, optimizing network usage is crucial:
- Minimize Data Transfer: Minimize the content that transmits over a network, or in other words, minimize the network traffic. Compress data where possible.
- Persistent Connections: As a best practice, avoid opening many connections when writing the request because it will cost time, use ‘Persistent Connections’ instead.
- Profiling and Continuous Monitoring Continuous performance monitoring and profiling can help in maintaining optimized code:Continuous performance monitoring and profiling can help in maintaining optimized code:
Monitoring Tools: To monitor the performance of your application you can use tools such as New Relic, Dynatrace and AWS CloudWatch.
Automated Profiling: Profiling should be run during CI/CD to detect performance degradation at the earliest possible, or at least during the development stage.
Conclusion
Performance tuning in code is a complex procedure where one must consider factors such as code’s efficiency, cohesion, and comprehensibility while being kept on the background of the overarching goal of the program in question. Thus, the utilization of accurate algorithms and data structures, the proper management of I/O operations, memory, and the application of modern compiler optimizations allows developers to work on and create applications with better performance. And finally, it is necessary to underline an importance of measuring the performance before and after the modifications, and never let oneself to be tricked into the optimization fallacy. In this way, profiling and monitoring can be done continuously to keep checking the continually changing code for efficiency.